
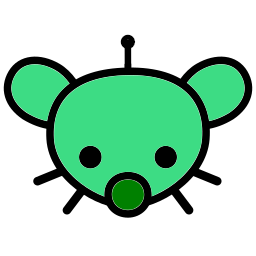
could try a flask webapp
from flask import Flask, send_from_directory
import os
app = Flask(__name__)
# Specify the directory you want to share
SHARED_DIRECTORY = 'shared_files'
@app.route('/files/<path:filename>', methods=['GET'])
def get_file(filename):
"""Serve a file from the shared directory."""
try:
return send_from_directory(SHARED_DIRECTORY, filename)
except FileNotFoundError:
return "File not found", 404
@app.route('/')
def list_files():
"""List files in the shared directory."""
files = os.listdir(SHARED_DIRECTORY)
files_list = '\n'.join(files)
return f"<h1>Files in {SHARED_DIRECTORY}</h1><pre>{files_list}</pre>"
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
I’m not seeing too many buzzwords here
https://grizzlyreports.com/we-believe-pdd-is-a-dying-fraudulent-company-and-its-shopping-app-temu-is-cleverly-hidden-spyware-that-poses-an-urgent-security-threat-to-u-s-national-interests/